Build Applications with
Fully Homomorphic
Encryption (FHE)
Zama is an open source cryptography company building state-of-the-art FHE solutions for blockchain and AI.
or read Zama's 6 minute intro to FHE.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.

Read Zama's latest posts on our blog.
Homomorphic encryption enables applications to run privately by processing data blindly.
.png)
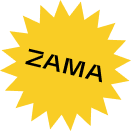

Write python code, run it on encrypted data
Zama's Concrete Framework enables data scientists to build models that run on encrypted data, without learning cryptography. Just write Python code and Concrete will convert it to an homomorphic equivalent!
Use low-level FHE operators to fine-tune execution
Cryptographers looking to manipulate FHE operators directly can do so using Concrete’s low-level library. Built in Rust using a highly modual architecture, it makes extending Concrete safe and easy.
FHE Brings Privacy to Web2 and Web3 Applications
And unlocks a myriad of new use cases.